Visualizing Stock Market Trends: Create a Sector Heat Map Using FMP API and Matplotlib
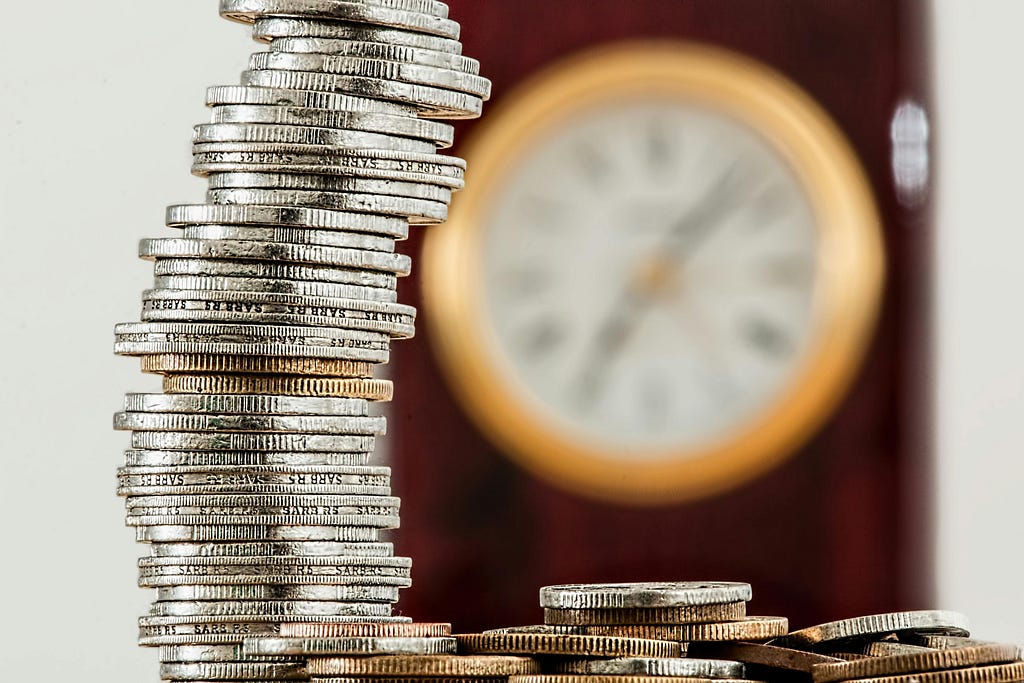
Stock market sectors move differently based on economic conditions, industry trends, and investor sentiment. Some sectors perform well during a market rally, while others decline. Tracking sector performance helps traders and investors understand which industries are gaining strength and which are losing momentum.
A sector heat map provides a quick visual representation of market trends. It highlights top-performing and underperforming sectors in a color-coded format. Instead of scanning through multiple stock charts, a heat map allows you to see market movements at a glance.
Manually analyzing sector data takes time and effort. You have to check multiple data sources and compare percentage changes for each sector. Automating this process makes it easier to track sector movements in real-time.
Financial Modeling Prep provides an API that fetches real-time sector performance data. With Python, we can process this data and create a heat map using Matplotlib. This allows us to quickly identify sector trends and make informed market decisions.
This article will cover how to fetch sector data, process it using Pandas, and visualize it as a heat map. By the end, you will have an automated system to track and analyze sector performance efficiently.
To create a sector heat map, we first need real-time sector performance data. Financial Modeling Prep provides an API that tracks the daily percentage change for each sector. This allows us to see which sectors are gaining or losing value.
The API returns data for multiple sectors, including technology, healthcare, financials, and energy. Each sector’s performance is expressed as a percentage change from the previous trading day. Positive values indicate growth, while negative values indicate a decline.
To access this data, we send a request to the FMP Sector Performance API. The API endpoint is:
https://financialmodelingprep.com/api/v4/sector-performance?apikey=YOUR_API_KEY
The response contains sector names and their percentage changes. Here’s a sample output:
{
"Technology": 2.5,
"Healthcare": -1.2,
"Financials": 0.8,
"Energy": -2.3
}
Now, let’s fetch this data using Python and convert it into a Pandas DataFrame.
import requests
import pandas as pd
# Define API Key
API_KEY = "YOUR_API_KEY"
URL = f"https://financialmodelingprep.com/api/v4/sector-performance?apikey={API_KEY}"
# Fetch data
response = requests.get(URL)
data = response.json()
# Convert to DataFrame
df = pd.DataFrame(list(data.items()), columns=["Sector", "Performance"])
df.set_index("Sector", inplace=True)
# Display the data
print(df)
This script retrieves the latest sector performance data and structures it in a table format. The DataFrame makes it easier to process and visualize the data.
Before creating the heat map, we will clean and format the data. This includes handling missing values and sorting sectors based on performance.
# Remove any missing values
df.dropna(inplace=True)
# Sort sectors by performance
df = df.sort_values(by="Performance", ascending=False)
print(df)
At this stage, we have a clean and structured dataset that is ready for visualization.
Creating a Sector Heat Map with Matplotlib
Now that we have processed the sector performance data, we will create a sector heat map using Matplotlib. A heat map helps visualize which sectors are performing well and which are underperforming.
Matplotlib provides a simple way to create a heat map by using the imshow() function, which colors each sector based on its percentage change. Positive values (gainers) will be shown in green, while negative values (losers) will be shown in red.
1. Install Matplotlib (If Not Installed)
If you haven’t installed Matplotlib, install it using:
pip install matplotlib
2. Plot the Sector Heat Map
We will now create a color-coded heat map for sector performance.
import matplotlib.pyplot as plt
import numpy as np
# Define colors (Green for gainers, Red for losers)
colors = ["green" if value > 0 else "red" for value in df["Performance"]]
# Create the heat map
plt.figure(figsize=(8, 6))
plt.barh(df.index, df["Performance"], color=colors)
# Add labels and title
plt.xlabel("Performance (%)")
plt.ylabel("Sectors")
plt.title("Stock Market Sector Performance Heat Map")
# Display the grid for reference
plt.grid(axis="x", linestyle="--", alpha=0.5)
# Show the heat map
plt.show()
This heat map ranks sectors based on performance, with the best-performing sectors at the top.
Free Stock Market API and Financial Statements API…
3. Adjusting the Heat Map for Better Visualization
If we want a deeper color gradient, we can use Seaborn for better styling.
pip install seaborn
import seaborn as sns
# Create a color gradient
plt.figure(figsize=(8, 6))
sns.heatmap(df.T, cmap="RdYlGn", annot=True, linewidths=0.5, fmt=".2f", cbar=True)
# Add title
plt.title("Stock Market Sector Heat Map")
plt.xlabel("")
plt.ylabel("")
# Show the heat map
plt.show()
This version uses a color gradient, making it easier to compare sector performance.
At this stage, we have successfully created a sector heat map that visually represents market trends.
Analyzing Market Trends with the Sector Heat Map
The sector heat map gives a quick visual representation of market trends. By analyzing it, we can identify which industries are performing well and which are struggling. This helps traders, investors, and analysts make informed decisions.
1. Identifying Strong and Weak Sectors
Sectors with green bars or positive values are leading the market, while those with red bars or negative values are underperforming.
To find the best and worst-performing sectors, we can check the top and bottom values in our dataset:
# Find the best-performing sector
best_sector = df.idxmax()["Performance"]
best_value = df.max()["Performance"]
print(f"Best Performing Sector: {best_sector} ({best_value:.2f}%)")
# Find the worst-performing sector
worst_sector = df.idxmin()["Performance"]
worst_value = df.min()["Performance"]
print(f"Worst Performing Sector: {worst_sector} ({worst_value:.2f}%)")
Interpretation:
- A strong technology sector may indicate investor confidence in innovation and growth stocks.
- A weak energy sector may reflect falling oil prices or regulatory concerns.
2. Understanding Sector Correlations
Some sectors move together, while others behave independently. For example, when technology stocks rise, they might pull up other growth sectors like consumer discretionary. If financials decline, it could indicate economic concerns.
We can compare sector performance over multiple days to identify correlations:
# Fetch sector data for multiple days (extend the API request)
historical_data = pd.DataFrame([
{"Sector": "Technology", "Performance": 2.5, "Date": "2024-03-01"},
{"Sector": "Technology", "Performance": 1.8, "Date": "2024-03-02"},
{"Sector": "Financials", "Performance": -1.2, "Date": "2024-03-01"},
{"Sector": "Financials", "Performance": -0.5, "Date": "2024-03-02"}
])
# Pivot for better visualization
pivot_df = historical_data.pivot(index="Date", columns="Sector", values="Performance")
# Plot the trend over time
pivot_df.plot(kind="line", figsize=(8, 5), marker="o", title="Sector Performance Over Time")
plt.xlabel("Date")
plt.ylabel("Performance (%)")
plt.grid()
plt.show()
Key Insights:
- If a sector performs well for multiple days, it may indicate sustained strength.
- A sector moving in the opposite direction of the market may signal a shift in investor sentiment.
3. Comparing Current Performance to Historical Trends
We can compare today’s sector performance against historical averages to spot unusual activity.
# Calculate average performance over time (assuming we have multiple days of data)
df["Historical_Average"] = df["Performance"].rolling(5).mean()
# Compare current vs. average
df["Above_Average"] = df["Performance"] > df["Historical_Average"]
print(df[["Performance", "Historical_Average", "Above_Average"]])
Why this matters?
- If a sector suddenly jumps above its average, it might indicate a new trend.
- If a sector falls below its usual performance, it could be due to negative news or economic shifts.
Key Takeaways from the Sector Heat Map
- Top-performing sectors indicate where money is flowing in the market.
- Sector correlations help understand market-wide trends.
- Historical comparisons reveal whether sector movements are temporary or long-term trends.
Conclusion
Conclusion
A sector heat map provides a clear and quick way to understand stock market trends. Instead of manually checking sector movements, we used Financial Modeling Prep (FMP) API to fetch real-time data and visualized it using Matplotlib. This makes it easy to see which industries are gaining momentum and which are lagging.
By automating sector analysis, traders and investors can quickly spot opportunities and risks. We processed the data using Pandas, created a heat map, and analyzed trends over time. This approach helps in making better investment decisions based on real-time sector performance.
This system can be expanded further by tracking historical trends, adding alerts for sector breakouts, or integrating more financial indicators. Automating market analysis saves time and provides valuable insights that manual tracking cannot match.
With this setup, you now have an automated tool to visualize and analyze sector performance efficiently.
Creating a Sector Heat Map with FMP and Matplotlib was originally published in Coinmonks on Medium, where people are continuing the conversation by highlighting and responding to this story.
Leave a comment